VA Forms Library - How to work with transformForSubmit
Sometimes you may have data that you want to reshape or adapt before sending it to the backend. The Forms Library manages this scenario by using an option called transformForSubmit
.
Options | Value Type | Description | Example |
---|---|---|---|
transformForSubmit | function | Called during form submission to transform submitted schema data (Optional). Calling transformForSubmit in platform/forms-system/src/js/helpers will remove view fields from the schema data. |
|
transformForSubmit
is an optional top level property on the formConfig object. If no custom transformForSubmit
function is provided, then the default transformForSubmit is run.
Example 1
In this example from the COVID-VACCINE-TRIAL Form, the following data has been transformed before being submitted to the backend: HEALTH_HISTORY
, TRANSPORTATION
, EMPLOYMENT_STATUS
, VETERAN
, GENDER
and RACE_ETHNICITY
.
Note: The image below shows only the GENDER
and RACE_ETHNICITY
sections of the form.
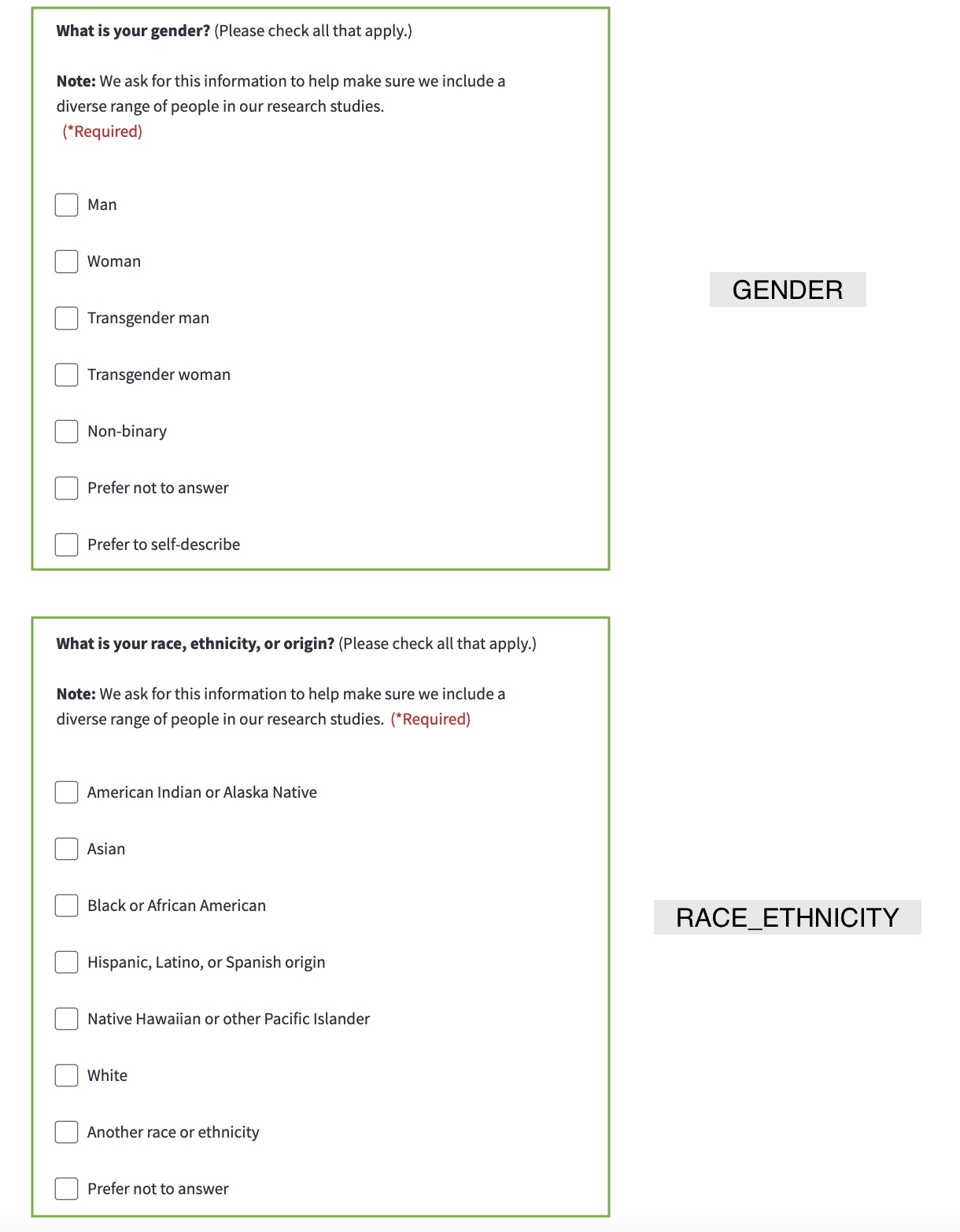
From the transform
function of COVID-VACCINE-TRIAL Form:
const checkBoxElements = [
'HEALTH_HISTORY',
'TRANSPORTATION',
'EMPLOYMENT_STATUS',
'VETERAN',
'GENDER',
'RACE_ETHNICITY',
];
export function transform(formConfig, form) {
const transformedForm = form;
checkBoxElements.forEach(elementName => {
Object.keys(form.data[elementName])
.filter(key => form.data[elementName][key] === undefined)
.forEach(filteredKey => {
transformedForm.data[elementName][filteredKey] = false;
});
});
return transformForSubmit(formConfig, transformedForm);
}
In this example, we transform the data of each item in the checkBoxElements
that is not selected (undefined
) to false
before sending it to the backend.
Example 2
In this example from the 10182 Form, which is used to request a Board Appeal, we reshape the form data before submitting it to the backend.
import {
addIncludedIssues,
addAreaOfDisagreement,
addUploads,
getAddress,
getPhone,
getTimeZone,
} from '../utils/submit';
export function transform(formConfig, form) {
// https://dev-developer.va.gov/explore/appeals/docs/decision_reviews?version=current
// https://github.com/department-of-veterans-affairs/vets-api/blob/master/modules/appeals_api/config/schemas/10182.json
const mainTransform = formData => {
const result = {
data: {
type: 'noticeOfDisagreement',
attributes: {
veteran: {
homeless: formData.homeless || false,
address: getAddress(formData),
phone: getPhone(formData),
emailAddressText: formData.veteran?.email || '',
},
boardReviewOption: formData.boardReviewOption || '',
hearingTypePreference: formData.hearingTypePreference || '',
timezone: getTimeZone(),
socOptIn: true,
},
},
included: addAreaOfDisagreement(addIncludedIssues(formData), formData),
nodUploads: addUploads(formData),
};
if (formData.boardReviewOption !== 'hearing') {
delete result.data.attributes.hearingTypePreference;
}
return result;
};
// Not using _.cloneDeep on form data - it appears to replace `null` values
// with an empty object; and causes submission errors due to type mismatch
const transformedData = mainTransform(form.data || {});
return JSON.stringify(transformedData);
}
In both examples, the transform
is called using the option transformForSubmit
in the formConfig file:
...
import { transform } from '../config/submit-transformer';
...
const formConfig = {
...
transformForSubmit: transform,
};
Help and feedback
Get help from the Platform Support Team in Slack.
Submit a feature idea to the Platform.