VA Forms Library - How to implement a personal information component populated with read-only data
Last Updated: April 23, 2025
This page provides guidance on implementing the personal information component within form applications, covering installation, usage, customization through configuration, and implementation examples. The component serves as a verification step at the beginning of form workflows, allowing users to confirm their personal information before proceeding with the rest of the form.
Features
The personal information component is a reusable React component used in VA.gov form applications to display a page that is used to validate a user's personal information. This page, sometimes referred to as a Veteran information page, typically appears at the beginning of form applications to confirm basic user information prefilled from the VA Profile data stored in Redux state and the in_progress_forms
endpoint.
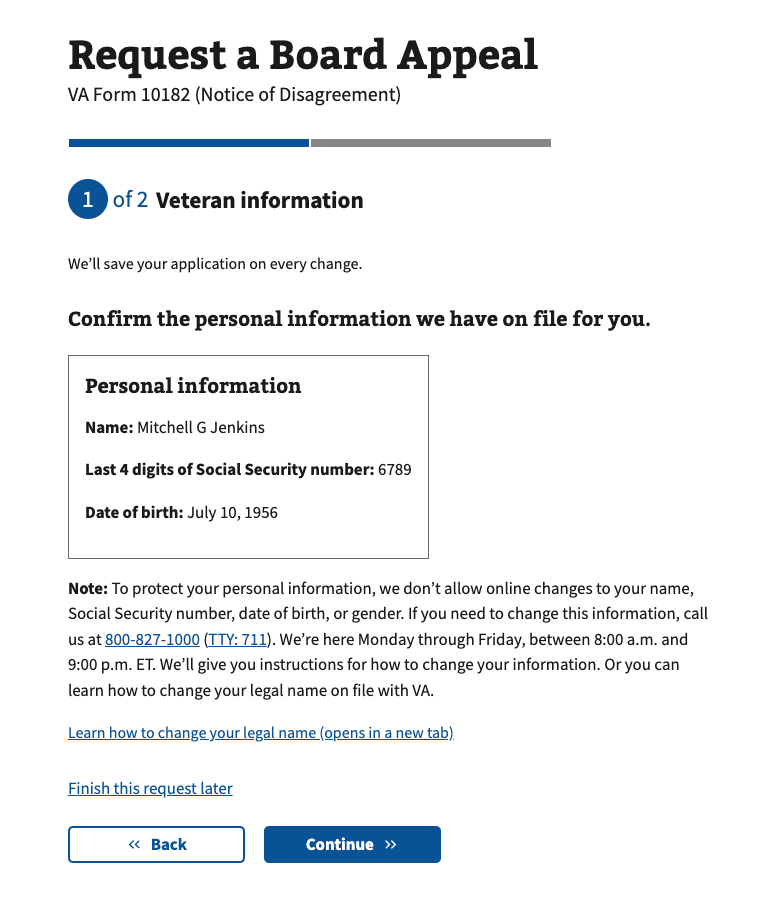
A personal information component populated with read-only data
Below is a list of the features of the personal information component:
Displays a user's personal information in a card format.
Configurable fields (name, SSN, VA file number, date of birth, sex).
Flexible data adaptation through configurable path lookups.
Accessibility support with screen reader formatting.
Navigation button integration.
Customizable error messages for missing required data (optional).
Custom header, footer, and note sections (optional).
Installation
This component is part of the VA.gov Forms system, so it should be available in your application via a direct import from the platform/forms-system
.
// import of the forms-system factory function that will provide a pre-built page based on configuration
import { personalInformationPage } from 'platform/forms-system/src/js/components/PersonalInformation';
// import of a CustomPage style component that can be further customized in rare circumstances by form teams
import { PersonalInformation } from 'platform/forms-system/src/js/components/PersonalInformation';
Usage
Basic implementation
Defaults to display name, SSN, and dateOfBirth.
Defaults to no fields being required for form to continue.
Assumes Redux state includes form data
form.data.ssn
.Assumes Redux state includes the profile data
user.prfoline.userFullName
anduser.profile.dob
.
import { personalInformationPage } from '@department-of-veterans-affairs/forms-system';
const formConfig = {
chapters: {
personalInformation: {
pages: {
...personalInformationPage()
}
}
}
};
Custom configuration
Key: allows the page to be uniquely identified in the form config.
Title: page title displayed in the page header.
Path: URL path for the page.
personalInfoConfig: allows field display and required status to be customized.
dataAdapter: allows data adapter paths to be customized, for when the prefill endpoint does not return the data in the expected format.
const customConfig = {
key: 'customPersonalInfo',
title: 'Veteran Information',
path: 'veteran-info',
personalInfoConfig: {
name: { show: true, required: true },
ssn: { show: true, required: true },
vaFileNumber: { show: true, required: false },
dateOfBirth: { show: true, required: true },
sex: { show: false, required: false }
},
dataAdapter: {
ssnPath: 'veteran.ssn',
vaFileNumberPath: 'veteran.vaFileNumber'
}
};
// application formConfig
const formConfig = {
chapters: {
personalInformation: {
pages: {
...personalInformationPage(customConfig)
}
}
}
};
Custom components
import {
PersonalInformation,
PersonalInformationNote,
PersonalInformationHeader,
PersonalInformationFooter,
PersonalInformationCardHeader
} from '@department-of-veterans-affairs/forms-system';
const CustomPersonalInfo = (props) => (
<PersonalInformation {...props}>
<PersonalInformationHeader>
<h2>Custom Header</h2>
</PersonalInformationHeader>
<PersonalInformationCardHeader>
<h3>Personal Details</h3>
</PersonalInformationCardHeader>
<PersonalInformationNote>
<p>Custom note content</p>
</PersonalInformationNote>
<PersonalInformationFooter>
<p>Custom footer content</p>
</PersonalInformationFooter>
</PersonalInformation>
);
Configuration options
PersonalInformationConfig
Controls the visibility and requirement status of each field.
interface FieldConfig {
show: boolean; // Whether to display the field
required: boolean; // Whether the field is required
}
interface PersonalInformationConfig {
name?: FieldConfig;
ssn?: FieldConfig;
vaFileNumber?: FieldConfig;
dateOfBirth?: FieldConfig;
sex?: FieldConfig;
}
DataAdapter
Configures paths to data in the form state.
interface DataAdapter {
ssnPath?: string; // Path to SSN in form data
vaFileNumberPath?: string; // Path to VA file number in form data
}
Data requirements
The page potentially expects data from two sources, the Redux state and the form data.
Redux state (VA Profile data):
userFullName
(first, middle, last, suffix)dob
(ISO format date string)gender
(is used to display the sex as Male or Female)
Form data (through data adapter):
SSN (last four digits)
VA File Number (last four digits)
Error handling
If data is missing from a required field, the component displays an error message, using either the default error component (DefaultErrorMessage
) or a custom error component passed through configuration. A required field not being filled is deemed fatal, and the form will not allow the user to continue to the next page as long as the error message is displayed. Whenever possible, provide a way to enter the missing data.
Security considerations
To ensure the security of a Veteran’s PII, the following considerations should be taken into account when creating personal information forms:
The form should display only the last four digits of SSN or VA File Number.
Personal information should not be directly editable. Instead, include a secure channel (i.e., a secure phone number) users can use to update the information in the form.
Best practices
Data adaptation
// Prefer using data adapter over direct prop passing
const adapter = {
ssnPath: 'veteran.ssn',
vaFileNumberPath: 'veteran.vaFileNumber'
};
Error handling
// Custom error component
const CustomError = ({ missingFields }) => (
<div className="custom-error">
{missingFields.map(field => (
<p key={field}>{`Missing ${field}`}</p>
))}
</div>
);
Component composition
// Use provided wrapper components for custom content if needing to build a CustomPage style component
<PersonalInformationNote>
<CustomNote />
</PersonalInformationNote>
Troubleshooting
Common issues
Below are some common problems and their solutions.
Missing data
If data is missing, verify that the Redux state contains VA Profile data. Make sure that data adapter paths match form state structure from the prefill endpoint and that the 'in_progress_forms/YOUR_FORM_NAME' endpoint is being called.
Display issues
If you encounter display issues, ensure that VA Design System components are properly imported and verify that CSS dependencies are included.
Navigation issues
If you encounter navigation issues, confirm that the NavButtons
component is properly passed and verify that goBack
and goForward
functions are provided.
Debugging
Using React DevTools
Enable React DevTools to inspect component properties and state changes.
Using Redux DevTools
Enable Redux DevTools to inspect the VA Profile state and the Form data state.
Contributing
When modifying this component, keep in mind the following:
Maintain accessibility standards.
Update tests for new features.
Document API changes.
Follow VA Design System guidelines, including using utility classes and web components.
Related documentation
Help and feedback
Get help from the Platform Support Team in Slack.
Submit a feature idea to the Platform.