React Router Guidelines
Last Updated:
These guidelines are aimed at realizing consistent, optimal router usage across all VFS apps. They’re also useful when upgrading an app from a previous version of react-router.
Modify component and hook imports
In the past we imported router functionality from react-router
. This functionality has been improved and moved to a new location. Import from react-router-dom
instead:
import { Switch, Route, Link, useHistory, useLocation } from 'react-router-dom';
Note that these new imports require a context to function. In unit tests you can provide the context by wrapping the component under test with a router component, as described in the react-router testing guide.
Use the new startApp()
function
The standard pattern for VSP apps is to call the startApp()
function to bootstrap the app. However, the new components and hooks described above are not compatible with the old startApp() function in platform/startup/index.js
. Instead, import the new startApp() function from platform/startup/router.js
. It’s a drop-in replacement.
Define routes using components
Instead of defining routes with a JS object, use react-router-dom
components. Here’s an example:
<Switch>
<Route path="/first">
<FirstComponent />
</Route>
<Route path="/second">
<SecondComponent />
</Route>
</Switch>
Define child routes inside child components. In this example, if FirstComponent
renders multiple child routes, those child routes should be defined inside FirstComponent
.
Avoid the render
and component
props where possible
Instead of placing your component inside the <Route render=...>
or <Route component=...>
props, just make your component a child of the Route component, like:
<Route path="/mypath">
<MyComponent exampleProp={someValue} />
</Route>
This method is cleaner because you explicitly pass any props your component needs.
Use hooks instead of withRouter
In older react-router
versions, it was common to use the withRouter
HOC to access router information or perform navigation. That approach is deprecated. It’s been replaced with simple hooks like these:
import { useParams, useLocation, useHistory } from 'react-router-dom';
const params = useParams();
const location = useLocation();
const history = useHistory();
history.push(`${location.pathname}/more/${params.id}`);
The react-router documentation lists supported hooks with example usage.
Handle unknown URLs by displaying a page not found
Handle unknown URL paths that fall within your application’s root URL by setting a default route that displays a page not found. This will provide the same 404 not-found-error user experience as for the static pages. This default route will trigger when no other routes are matched, so care must be taken to specify your valid route paths as detailed as possible to catch all instances of unknown paths. In the sample below, a path of /first/dummy will display a page not found because the path does not match any routes (note the first route only matches /first as it is exact), but a path of /second/dummy will route to the SecondComponent since that route is not exact.
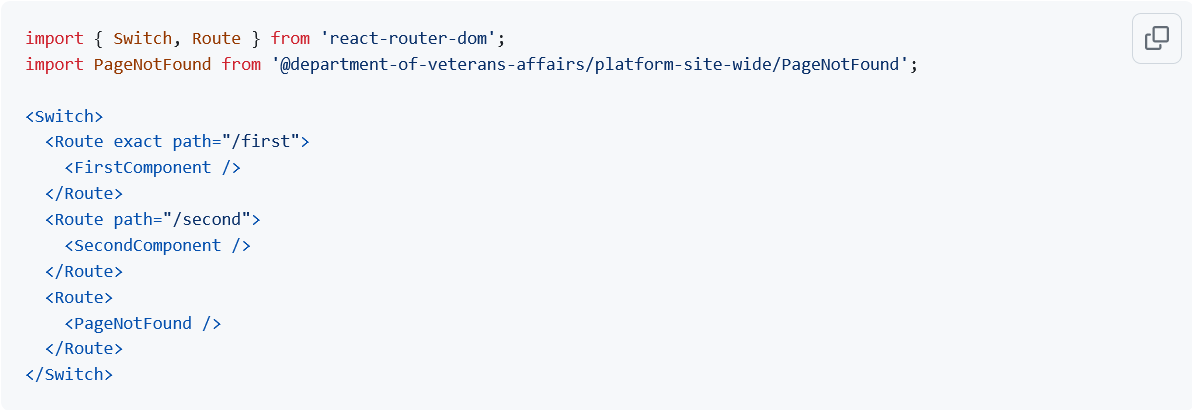
Note: You need to render the PageNotFound component by itself (e.g. no breadcrumbs, side navigation, etc.) for the proper user experience.
Help and feedback
Get help from the Platform Support Team in Slack.
Submit a feature idea to the Platform.