Checking if an uploaded PDF is encrypted
Last Updated: April 21, 2025
This page contains instructions on how to add a password field to the va-file-input
web component to an uploaded encrypted PDF.
Why is this necessary?
The va-file-input
web component does not check if a file is encrypted. You will need to submit a password along with it. The Platform provides utilities to perform this check.
Step-by-step guide
The following steps require you to use Platform utilities.
STEP #1: Import the React binding to make it easier to handle event callbacks.
import { VaFileInput } from '@department-of-veterans-affairs/web-components/react-bindings';
STEP #2: Import the utility functions from the platform.
import {
readAndCheckFile,
checkIsEncryptedPdf,
} from 'platform/forms-system/src/js/utilities/file';
STEP #3: Set up a function to handle the state.
This example uses React’s useState
, but state management is up to you.
import React, { useState } from 'react';
const [showPasswordInput, setShowPasswordInput] = useState(false);
async function checkIfEncrypted(event) {
const file = event.detail.files[0];
if (file) {
// only run the check if the file exists
const checkResults = await readAndCheckFile(file, {
checkIsEncryptedPdf,
});
setShowPasswordInput(checkResults.checkIsEncryptedPdf);
}
}
In this example, checkIfEncrypted
is the callback on onVaChange
. The function passes the file and checkIsEncryptedPdf
to readAndCheckFile
, which returns an object with an boolean attribute checkIsEncryptedPdf
.
STEP #4: Once showPasswordInput
is set, you can pass it to the encrypted
attribute of va-file-input
to show a password field.
<VaFileInput onVaChange={checkIfEncrypted} encrypted={showPasswordInput} />
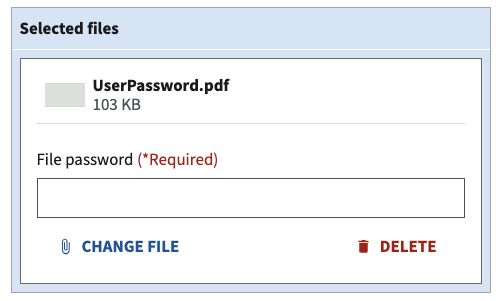
The va-file-input component with the file password field revealed
FINAL CODE: This is what the final code should look like.
import React, { useState } from 'react';
import { VaFileInput } from '@department-of-veterans-affairs/web-components/react-bindings';
import {
readAndCheckFile,
checkIsEncryptedPdf,
} from 'platform/forms-system/src/js/utilities/file';
export default function FileInputWithPassword() {
const [showPasswordInput, setShowPasswordInput] = useState(false);
async function checkIfEncrypted(event) {
const file = event.detail.files[0];
if (file) {
// only run the check if the file exists
const checkResults = await readAndCheckFile(file, {
checkIsEncryptedPdf,
});
setShowPasswordInput(checkResults.checkIsEncryptedPdf);
}
}
return (
<VaFileInput onVaChange={checkIfEncrypted} encrypted={showPasswordInput} />
);
}
Help and feedback
Get help from the Platform Support Team in Slack.
Submit a feature idea to the Platform.